C Program to check the number is a leap year or not?
Problem:
Write a C program to check if the number is a leap year or not.
Solution:
Logic for a number is leap year or not:
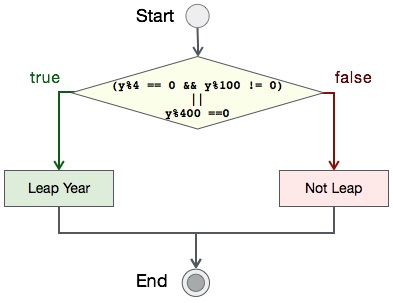
C code to check leap year Using simple if-else
#include <stdio.h>
int main()
{
int year;
printf("Enter a year to check if it is a leap year\n");
scanf("%d", &year);
if ( year%400 == 0)
printf("%d is a leap year.\n", year);
else if ( year%100 == 0)
printf("%d is not a leap year.\n", year);
else if ( year%4 == 0 )
printf("%d is a leap year.\n", year);
else
printf("%d is not a leap year.\n", year);
return 0;
}
C Code to check leap year More accurate with advance nesting if-else
#include <stdio.h>
int main()
{
int year;
printf("Enter a year: ");
scanf("%d",&year);
if(year%4 == 0)
{
if( year%100 == 0)
{
// year is divisible by 400, hence the year is a leap year
if ( year%400 == 0)
printf("%d is a leap year.", year);
else
printf("%d is not a leap year.", year);
}
else
printf("%d is a leap year.", year );
}
else
printf("%d is not a leap year.", year);
return 0;
}
Demonstration How to check if the number is leap year or not : C code implementation:
[Demonstrating the advance one]
[Demonstrating the advance one]
- Come to logic: We know that a leap year is divisible by 4 and if that is divisible by 100, then it will also divisible by 400.
- And now check the c code and hopefully you can check the code now.
Tags:
C Program to check the number is a leap year or not? Leap year check, check leap year c code, leap year code.
Hey thank you for providing this program. I have related one visit leap year program
ReplyDelete